Triggered Notifications (Events)
Events are user interactions with content on your page. These are independent of website load and allow you to track the behavior of visitors. Examples of custom events can be a download, link click, scroll to a particular part of page, video play, ad-click and so on.
A single page on your website can trigger multiple events based on the subscriber's interaction.
Parts of an Event
An event can be defined using 4 pieces of information out of which two are compulsory.
- eventCategory - string or integer (Required)
- eventAction - string or integer (Required)
- eventLabel - string or integer (Optional)
- eventValue - integer (Optional)
How to create and track a custom event?
Step 1: Creating a custom event
To start with custom event tracking, you need to first define it in your PushAlert dashboard. Here are the steps:
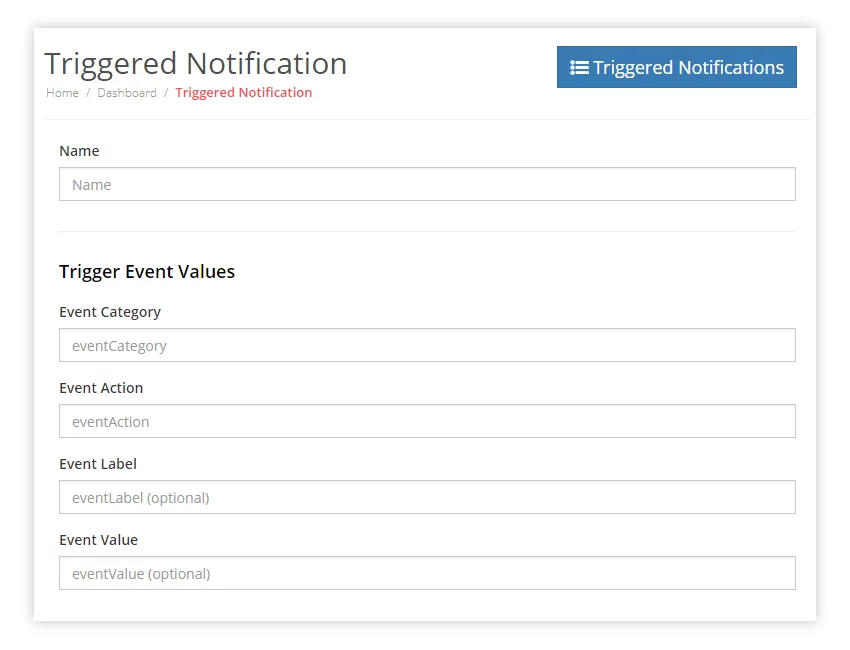
- Head to Automation > Triggered Notifications on your PushAlert Dashboard.
- Create a new Triggered Notification.
- Fill in the name of the event, this will be useful for searching through created events.
- Add the eventCategory, eventAction parameters that you want tracked, optionally you can also add the eventLabel and eventValue parameters as well.
- Once done, the next step is to configure the notification that is send when the created event is triggered.
- Fill in the Title, Message and the link you want the subscriber to land on. You can also change the image, add action buttons and set an expiry time.
- Specify the delay after which you want the notification to be triggered after the event has successfully completed (in multiples of 5).
Step 2: Adding events to website
There are two ways you can add and track events on your website:
Using JavaScript API
Add the following script on the page where you want to track an event. You can track multiple events on the same page.
Request Parameters
Parameter | Type | Description |
---|---|---|
eventCategory | String or Integer | Required The object or page that was interacted with (e.g. 'Video'). |
eventAction | String or Integer | Required The type of interaction (e.g. 'play','pause','stopped'). |
eventLabel | String or Integer | Optional Used for categorizing events. (e.g. 'Launch Campaign'). |
eventValue | Integer | Optional A numeric value associated with the event (e.g. 2017). |
Example
(pushalertbyiw = window.pushalertbyiw || []).push(['trackEvent', 'video', 'play', 'winter collection', '51']); //trackEvent(eventCategory, eventAction, eventLabel, eventValue)
Using REST API
POST /rest/v1/track/event
Using this endpoint you can trigger a notification in the back-end based on user interaction. It requires the subscription ID to be passed along with the event parameters.
Resource Information
Method | POST |
---|---|
URL | https://api.pushalert.co/rest/v1/track/event |
Requires authentication? | Yes |
Request Parameters
Parameter | Type | Description |
---|---|---|
subscriber | String | Required The subscriber ID for the subscriber that triggered the event. |
eventCategory | String or Integer | Required The object or page that was interacted with (e.g. 'Video'). |
eventAction | String or Integer | Required The type of interaction (e.g. 'play','pause','stopped'). |
eventLabel | String or Integer | Optional Used for categorizing events. (e.g. 'Launch Campaign'). |
eventValue | Integer | Optional A numeric value associated with the event (e.g. 2017). |
Example
curl https://api.pushalert.co/rest/v1/track/event \
-H "Authorization: api_key=<insert api key here>" \
-d "subscriber=SUBSCRIBER_ID&eventCategory=video&eventAction=play&eventLabel=winter%20collection&eventValue=51"
<?php
$subscriber = "SUBSCRIBER_ID";
$eventCategory = "video";
$eventAction = "play";
$eventLabel = "winter collection";
$eventValue = "51";
$apiKey = "YOUR_API_KEY";
$curlUrl = "https://api.pushalert.co/rest/v1/track/event";
//POST variables
$post_vars = array(
"subscriber" => $subscriber,
"eventCategory" => $eventCategory,
"eventAction" => $eventAction,
"eventLabel" => $eventLabel,
"eventValue" => $eventValue,
);
$headers = Array();
$headers[] = "Authorization: api_key=".$apiKey;
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $curlUrl);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($post_vars));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$result = curl_exec($ch);
$output = json_decode($result, true);
if($output["success"]) {
echo "success";
}
else {
//Others like bad request
}
?>
Output
{
"success": true
}